How to Import and Export Functions in JavaScript
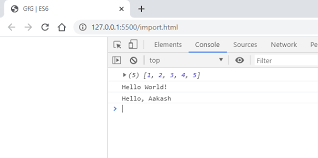
JavaScript is a versatile language that allows developers to create complex and dynamic web applications. One of the key features of JavaScript is its ability to import and export functions.
Importing and exporting functions allows developers to organize their code into separate modules, making it easier to manage and maintain. In this article, we will explore how to import and export functions in JavaScript.
Importing Functions
To import a function from another module, you first need to export the function from the module. You can export a function using the export statement, followed by the function definition:
“`
// myModule.js
export function myFunction() {
// function code goes here
}
“`
Once you have exported the function, you can import it into another module using the import statement:
“`
// main.js
import { myFunction } from ‘./myModule.js’;
myFunction();
“`
In this example, we are importing the `myFunction` from the `myModule.js` file using the `import` statement. We can then use the imported function as we would any other function.
It’s important to note that the file path in the `import` statement must be relative to the current file. If the file you are importing from is in the same directory, you can simply use the file name. If it is in a different directory, you will need to specify the path relative to the current file.
Exporting Functions
Exporting functions is similar to importing functions. To export a function, use the `export` statement before the function definition:
“`
// myModule.js
function myFunction() {
// function code goes here
}
export { myFunction };
“`
In this example, we are exporting the `myFunction` function using the `export` statement. We can then import this function into another module using the `import` statement discussed earlier.
You can also use the `export default` statement to export a default function or object from a module:
“`
// myModule.js
export default function() {
// default function code goes here
}
“`
When using `export default`, you can import the default function without the use of curly braces:
“`
// main.js
import myDefaultFunction from ‘./myModule.js’;
myDefaultFunction();
“`
In this example, we are importing the default exported function from `myModule.js` and using it as we would any other function.