How to Use the Java TreeMap Data Structure
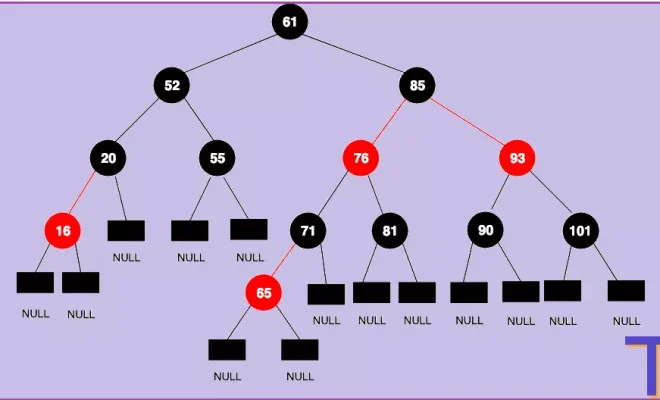
The TreeMap data structure in Java is one of the most commonly used data structures for sorting and associating values with keys. It’s based on the concept of a binary search tree, where each node in the tree has a key and a value associated with it.
In this article, we will show you how to use the Java TreeMap data structure, and we’ll walk you through the steps to create, modify, and iterate over the TreeMap.
1. Creating a TreeMap
The first step in using the TreeMap is to create a new instance of it. You can do this with the following code:
“`
TreeMap treeMap = new TreeMap();
“`
In the above example, we’re creating a TreeMap that uses Strings as keys and Integers as values. You can change the types of keys and values as per your requirements.
2. Adding Elements to the TreeMap
Once you’ve created a TreeMap, you can add elements to it using the `put()` method. The `put()` method takes two parameters – the key and the value.
Here’s an example:
“`
treeMap.put(“A”, 1);
treeMap.put(“B”, 2);
treeMap.put(“C”, 3);
“`
In the above example, we have added three elements to the TreeMap. Each element consists of a key-value pair.
3. Modifying Elements in the TreeMap
You can modify the values associated with a particular key by using the `put()` method again.
Here’s an example:
“`
treeMap.put(“A”, 5);
“`
In the above example, we’re modifying the value associated with the key “A”. The new value associated with the key “A” is now 5.
4. Removing Elements from the TreeMap
You can remove elements from a TreeMap by using the `remove()` method. The `remove()` method takes one parameter – the key of the element you want to remove.
Here’s an example:
“`
treeMap.remove(“A”);
“`
In the above example, we’re removing the element with the key “A” from the TreeMap.
5. Iterating Over the TreeMap
You can iterate over a TreeMap using a for-each loop. The for-each loop will iterate over each element in the TreeMap and print out the key-value pairs.
Here’s an example:
“`
for(Map.Entry entry : treeMap.entrySet()) {
System.out.println(entry.getKey() + ” ” + entry.getValue());
}
“`
In the above example, we’re iterating over all the elements in the TreeMap using the `entrySet()` method. The `entrySet()` method returns a set of all the key-value pairs in the TreeMap.
Conclusion
The TreeMap in Java is a powerful data structure that is used for sorting and associating values with keys. It’s easy to create, modify, and iterate over a TreeMap in Java. By following the steps outlined in this article, you should be able to use the TreeMap data structure in your Java programs with ease.