How Error Boundaries Work in React
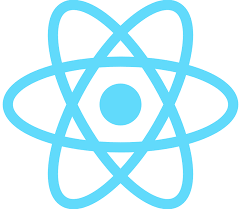
React is one of the most popular JavaScript frameworks, and one of its key features is its ability to handle errors in a graceful and efficient manner. One of the ways it does this is through the use of error boundaries. In this article, we will take a closer look at error boundaries and how they work in React.
What are Error Boundaries in React?
An error boundary is a component in React that is specifically designed to handle errors that occur within one of its child components. This component catches any errors that occur in its child component tree, logs the error, and displays a fallback UI rather than crashing the entire application.
The error boundary component is just like any other React component, with a few extra methods that allow it to handle errors. The two methods included in an error boundary component are static getDerivedStateFromError() and componentDidCatch().
How Do Error Boundaries Work in React?
Let’s take a look at the lifecycle of an error boundary component in React:
1. In the mounting phase, the component will display its child components as normal.
2. If an error occurs in one of the child components, the error boundary component will catch the error and call its componentDidCatch() method.
3. The componentDidCatch() method logs the error and sets a flag in the component state to indicate that an error has occurred.
4. The error boundary component re-renders itself, using the fallback UI provided in its render() method.
5. The fallback UI is displayed to the user, indicating that an error has occurred in the child component.
The fallback UI is typically a simple message to the user, indicating that an error has occurred, and that the application is being restored to a stable state. This allows the user to continue using the other parts of the application that are not affected by the error.
Benefits of Error Boundaries
The main benefit of error boundaries is that they prevent the entire application from crashing when an error occurs. This avoids the frustrating experience of the user seeing a blank screen or having to restart the application.
Additionally, error boundaries make it easier to debug errors in the application. By logging the error and providing a fallback UI, error boundaries give developers insight into where and why an error occurred, allowing them to quickly diagnose and fix the issue.
Conclusion
Error boundaries are a powerful tool in React, allowing developers to gracefully handle errors and prevent the entire application from crashing. By providing a fallback UI and logging errors, error boundaries make it easier to diagnose and fix errors, improving the user experience and overall stability of the application.