How to Write a Morse Code Translator in Python
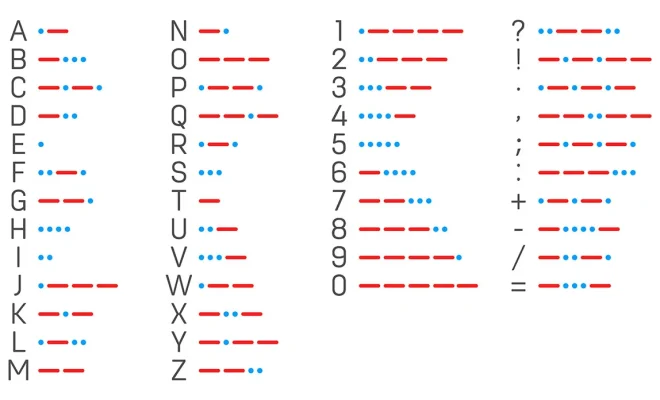
Morse code became one of the earliest forms of long-distance communication in the world. Developed in the 1830s and 1840s, it is a system of communication using dots and dashes to represent letters, numbers, and punctuation. With the development of radio communication in the 20th century, Morse code became an integral part of long-distance communication among pilots, sailors, and radio operators. Writing a Morse code translator in Python is relatively easy, and in this article, we will discuss the steps to create one.
- Understanding Morse Code
The first step in writing a Morse code translator in Python is to understand the Morse code. Morse code consists of dots and dashes, where the dots represent a short signal, and the dashes represent a long signal. Each letter, number, and punctuation mark is represented by a unique sequence of dots and dashes.
The International Morse code consists of 26 letters, 10 numbers, and some special characters, such as the period, comma, and question mark. You can find a table of the Morse code symbols online or in books.
- Creating a Python Dictionary
Once you understand the Morse code symbols, the next step is to create a Python dictionary that can translate the dots and dashes to their corresponding letters, numbers, and punctuation marks. To create a dictionary, use the curly braces {} and separate each key-value pair with a colon (:). Here is an example dictionary:
“`
morse_code = {
‘.-‘: ‘a’,
‘-…’: ‘b’,
‘-.-.’: ‘c’,
‘-..’: ‘d’,
‘.’: ‘e’,
‘..-.’: ‘f’,
‘–.’: ‘g’,
‘….’: ‘h’,
‘..’: ‘i’,
‘.—‘: ‘j’,
‘-.-‘: ‘k’,
‘.-..’: ‘l’,
‘–‘: ‘m’,
‘-.’: ‘n’,
‘—‘: ‘o’,
‘.–.’: ‘p’,
‘–.-‘: ‘q’,
‘.-.’: ‘r’,
‘…’: ‘s’,
‘-‘: ‘t’,
‘..-‘: ‘u’,
‘…-‘: ‘v’,
‘.–‘: ‘w’,
‘-..-‘: ‘x’,
‘-.–‘: ‘y’,
‘–..’: ‘z’,
‘—–‘: ‘0’,
‘.—-‘: ‘1’,
‘..—‘: ‘2’,
‘…–‘: ‘3’,
‘….-‘: ‘4’,
‘…..’: ‘5’,
‘-….’: ‘6’,
‘–…’: ‘7’,
‘—..’: ‘8’,
‘—-.’: ‘9’,
‘.-.-.-‘: ‘.’,
‘–..–‘: ‘,’,
‘..–..’: ‘?’,
‘-..-.’: ‘/’
}
“`
In the above example, each key is a string of dots and dashes, and each value is a letter, number, or punctuation mark.
- Writing the Code
With the dictionary created, writing the actual code for the Morse code translator is straightforward. Here is an example function that takes a string of dots and dashes as input and returns the corresponding text:
“`
def morse_to_text(morse_code, morse_string):
text = ”
words = morse_string.split(‘ / ‘)
for word in words:
letters = word.split(‘ ‘)
for letter in letters:
if letter in morse_code:
text += morse_code[letter]
text += ‘ ‘
return text
“`
The function takes two arguments – the Morse code dictionary and the Morse code string. The string is first split by the space character to get the individual words. Then, each word is split again by the ‘/’ character to separate the words. Finally, each letter is looked up in the dictionary, and the corresponding text is appended to the output string.
- Testing the Code
You can test the Morse code translator with some Morse code input, such as “.-. .-.. .- – / – .. … … – ..- –.”, which translates to “ralph must too”. To test the code, you can use the following lines:
“`
morse_code = {
# your dictionary here
}
morse_input = “.- .-.. .- — / — .. … … ..- -.”
text_output = morse_to_text(morse_code, morse_input)
print(text_output)
“`
When you run the above lines, you should see the output “ralph must too” on your screen.