Learn How to Create Classes in C#
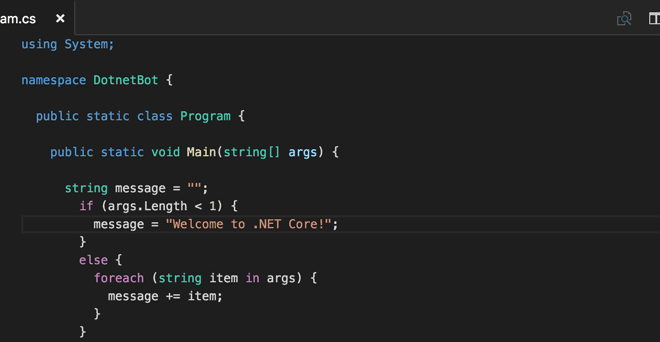
C# is an object-oriented programming language that allows developers to create classes. A class is a blueprint for creating objects, which are instances of the class. Classes allow developers to organize their code and create reusable code that can be used multiple times in their programs.
Learning how to create classes in C# is essential for anyone interested in becoming a C# developer. Classes are the building blocks of object-oriented programming, and understanding how they work will help you develop applications that are robust and scalable.
To create a class in C#, you first need to define the class using the class keyword. Here is an example of how to create a class:
“`
public class MyClass
{
// class members go here
}
“`
In this example, we have created a class called “MyClass” using the “public” access modifier. The access modifier determines the level of access other classes have to this class. Public classes can be accessed by any code in the program, while private classes can only be accessed within the same class.
After defining the class, you can add members to the class. Members are the data and behavior of the class. There are two types of members in C#: fields and methods.
Fields are variables that store data within the class. Here is an example of how to add a field to the “MyClass” class:
“`
public class MyClass
{
public string myField = “Hello, world!”;
}
“`
In this example, we have added a public field called “myField” that stores the string “Hello, world!”.
Methods are functions that perform operations within the class. Here is an example of how to add a method to the “MyClass” class:
“`
public class MyClass
{
public void MyMethod()
{
Console.WriteLine(“Hello, world!”);
}
}
“`
In this example, we have added a public method called “MyMethod” that writes the string “Hello, world!” to the console.
Once you have defined your fields and methods, you can create objects of the class. Here is an example of how to create an instance of the “MyClass” class:
“`
MyClass myObject = new MyClass();
“`
In this example, we have created an instance of the “MyClass” class called “myObject”. Now we can access the fields and methods of the class using the “myObject” instance.
“`
Console.WriteLine(myObject.myField); // output: Hello, world!
myObject.MyMethod(); // output: Hello, world!
“`
In conclusion, learning how to create classes in C# is essential for anyone interested in becoming a C# developer. Classes allow developers to organize their code and create reusable code that can be used multiple times in their programs. By understanding how to define classes, add members, and create objects, you will be able to create robust and scalable applications with C#.